This is my Unity Scriptbook. In it I’ll be writing scripts to be used for different purposes of all kinds, like a reference sheet. They range from complicated to extremely simple, but I’ve used each multiple time. I hope you find something useful here that will make your scripting a little easier.
Rotate 2d character towards mouse
Add or subtract degrees in the angle variable depending on your objects orientation
private void RotateTowardMouse() { // get mouse position Vector3 direction = target - transform.position; // determine new angle, subtract angles based on sprite orientation var angle = Mathf.Atan2(direction.y, direction.x) * Mathf.Rad2Deg - 90; // rotate gameobject Quaternion rotation = Quaternion.AngleAxis(angle, Vector3.forward); transform.rotation = rotation; }
Rotate a 2d gameobject toward target (any gameobject)
private void RotateEnemyTowardsPlayer(Transform target) { // get target position Vector3 direction = target.position - transform.position; // get player position, move and rotate towards that position var angle = Mathf.Atan2(direction.y, direction.x) * Mathf.Rad2Deg - 90; // rotate toward target Quaternion rotation = Quaternion.AngleAxis(angle, Vector3.forward); transform.rotation = rotation; }
How to filter out collisions in Physics2D settings
Layer based collision : You can filter collisions out, so for example if the player will only ever collide with the ‘Ground’ layer than you can make it so in the collision matrix. Here is an example where only certain layers are allowed to collide with each other. Should make the game a little more performant as it ignores collisions not highlighted in the collision matrix.
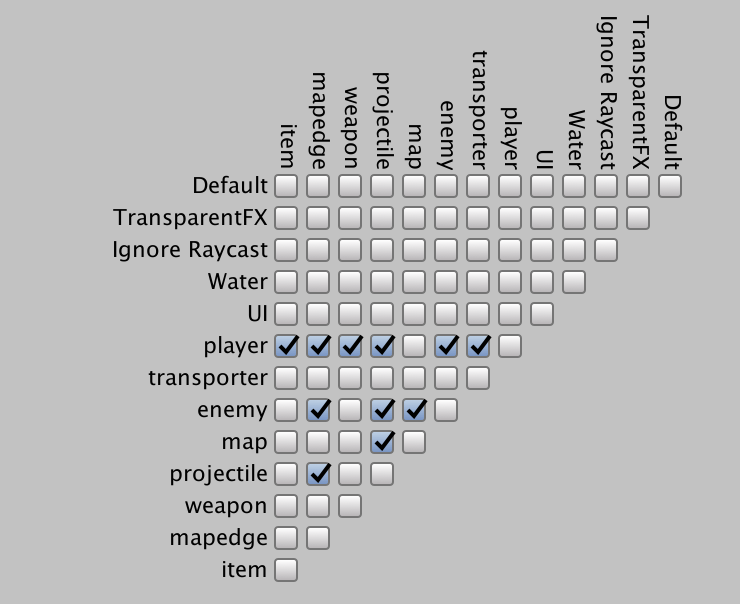
How to filter collisions out in code
If you do have multiple layers you will be colliding with then this code snippet can help in differentiating collisions out.
private void OnCollisionEnter2D(Collision2D collision) { if (collision.gameObject.layer != LayerMask.NameToLayer("enemy")) { // do something } }
Rotating an object around a point
You think you would use sin, and cos to find your position around a point, unity makes it easy and adds a function called RotateAround.
private void PlayerCircularMovement(float angleOnCircle, float anglesPerSecond) { float movement = Input.GetAxisRaw("Horizontal") * Time.deltaTime * anglesPerSecond; float newAngleOnCircle = (angleOnCircle + movement) % 360; // 1st parameter: point on which to rotate around // 2nd parameter: axis to rotate around // 3rd parameter: angle to rotate to transform.RotateAround(Vector3.zero, Vector3.forward, angleOnCircle); }
Finding child game objects
I don’t use this on to often but it can help if a parent gameobject needs to get to one of its children
aFinger = transform.Find("Hand/Gun");
or if you have multiple of the same name you can use the following
for (var child in transform) { if (child.name == "Gun") { // code is called for all guns, like fire } }
Easy pause functionality
private bool _isPaused; private void Pause() { if (_isPaused == true) { isPaused = false; Time.timeScale = 1; } else { isPaused = true; Time.timeScale = 0; } }
Easy restart funtionality
if (Input.GetKeyDown(KeyCode.Space)) { SceneManager.LoadScene(SceneManager.GetActiveScene().buildIndex); }
Mouse click to game world position
This simple conversion converts a screen point into a world point. You can then use the world point for a numerous amount of things. For example shooting a bullet in the mouse button direction
if (Input.GetMouseButtonDown(0)) { Vector2 position = (Vector2)cam.ScreenToWorldPoint(Input.mousePosition); }
2D Recoil Effect Script
This script adds recoil to the game object it is attached to. To add recoil in a specific direction you can create a function that takes a direction vector. ONE caveat when using this script is that the _maximumOffsetDistance WILL need to be reachable given your acceleration and starting speed, else it will wobble back and forth.
using UnityEngine; public class RecoilScript : MonoBehaviour { public float _maximumOffsetDistance; public float _recoilAcceleration; public float _weaponRecoilStartSpeed; private bool _recoilInEffect; private bool _weaponHeadedBackToStartPosition; private Vector3 _offsetPosition; private Vector3 _recoilSpeed; public void AddRecoil() { _recoilInEffect = true; _weaponHeadedBackToStartPosition = false; _recoilSpeed = transform.right * _weaponRecoilStartSpeed; } private void Start() { _recoilSpeed = Vector3.zero; _offsetPosition = Vector3.zero; _recoilInEffect = false; _weaponHeadedBackToStartPosition = false; } private void Update() { UpdateRecoil(); } private void UpdateRecoil() { if (_recoilInEffect == false) { return; } // calculate current speed given our acceleration and opposite in direction of our offset // basically we calculate the new velocity given acceleration, then we calculate the new position // given the new velocity _recoilSpeed += (-_offsetPosition.normalized) * _recoilAcceleration * Time.deltaTime; Vector3 newOffsetPosition = _offsetPosition + _recoilSpeed * Time.deltaTime; Vector3 newTransformPosition = transform.position - _offsetPosition; if (newOffsetPosition.magnitude > _maximumOffsetDistance) { _recoilSpeed = Vector3.zero; _weaponHeadedBackToStartPosition = true; newOffsetPosition = _offsetPosition.normalized * _maximumOffsetDistance; } else if (_weaponHeadedBackToStartPosition == true && newOffsetPosition.magnitude > _offsetPosition.magnitude) { transform.position -= _offsetPosition; _offsetPosition = Vector3.zero; // set up our boolean _recoilInEffect = false; _weaponHeadedBackToStartPosition = false; return; } transform.position = newTransformPosition + newOffsetPosition; _offsetPosition = newOffsetPosition; } }
2D Laserbeam Script
Add a laser beam to an object of your choice. See how to set it up here.
Creating a laser beam effect in Unity
using UnityEngine; public class LaserPointer : MonoBehaviour { public float _laserBeamLength; private LineRenderer _linerenderer; private void Start() { _linerenderer = GetComponent<LineRenderer>(); } private void Update() { Vector3 endPosition = transform.position + (transform.right * _laserBeamLength); _linerenderer.SetPositions(new Vector3[] { transform.position, endPosition }); } }